Home › Forums › Software Development › Checking whether if the eye tracker is connected › Reply To: Checking whether if the eye tracker is connected
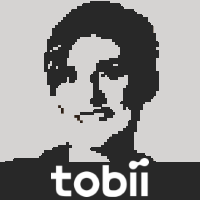
Hi!
Robert asked me to give you an example of how to retrieve the eye tracking device status. Normally, when the eye tracking device is connected to the computer and up and running, its status would be “Tracking”. If the user has selected “Disable Eye Tracking” in the tray icon menu, the status is normally “Tracking Paused”.
This is how you can retrieve information about the eye tracking device status through the .NET binding:
First, where all handlers are registered, register a notification handler and register the context as a settings observer as well:
context.RegisterNotificationHandler(HandleNotification);
context.RegisterSettingsObserver(SettingsPaths.EyeTracking);
Then, add methods for handling notification callbacks:
private void HandleNotification(InteractionNotification notification)
{
if (NotificationType.SettingsChanged == notification.NotificationType)
{
OnSettingsReceived((SettingsBag)notification.Data);
}
}
private void OnSettingsReceived(SettingsBag data)
{
EyeTrackingDeviceStatus deviceStatus;
var success = data.TryGetSettingValue(out deviceStatus, "eyetracking.state");
if (success)
{
Console.WriteLine("Eye Tracking Device Status is: " + deviceStatus);
}
}
Does that give you the information you need?